![]() |
Photo by Caleb Oquendo from Pexels |
Karate is a open source API test automation framework, it's an extension of cucumber framework which follows BDD. Since the cucumber syntax are very human readable even non programmers can understand/write tests easily. You define your test scenario in a Gherkin file which also know as a feature file. Karate has many more features that we can use to ease our API testing, this post will give you enough details to start implementing your own testing project.
Although we write unit tests to cover up the functionality of the code, having this type of integration tests puts you more confident to release a feature, patch to QA or even a pre-QA urgent release to production. Because having actual tests can make sure your code fix did not break anything.
What you will learn
- Write a simple feature file with test scenario and execute test
- JUnit 5 integration
- Some example with karate
- Reporting
Getting Started
We can use standard maven project to write our tests. Here in this post I'm using a spring-boot project because to run the example I have implemented a simple rest service. You need to add below two dependencies.
<dependency>
<groupId>com.intuit.karate</groupId>
<artifactId>karate-apache</artifactId>
<version>0.9.5</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.intuit.karate</groupId>
<artifactId>karate-junit5</artifactId>
<version>0.9.5</version>
<scope>test</scope>
</dependency>
We also need latest maven-surefire-plugin to support junit and reports
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.2</version> </plugin>
Follow Up
If you wish to follow up with this blog post using the sample project, please follow the below steps.
Technologies used
Technologies used
- Java 8
- Apache Maven 3.6.3
- JUnit 5
- Karate
- Spring-boot
1) Clone the project - https://github.com/Rajithkonara/API-test-karate-example
2) Open the project using your IDE. (I'm using Intellij idea)
3) Run the Spring-boot application. (Run the KarateApplication class)
The sample spring-boot application is exposing a mock REST service which we can use to do API testing with karate. While checking out karate documentation I found this Repo as a recommended example which uses JUnit 4. I also used this repo as a reference.
Folder Structure
Since karate scripts are non java files and usually these files should go to /src/test/resources directory, but karate recommends to keep these feature files alone side with java files. To allow that add below to build section in your pom file. Basically your tests should comes under /src/test/java directory
<build>
<testResources>
<testResource>
<directory>src/test/java</directory>
<excludes>
<exclude>**/*.java</exclude>
</excludes>
</testResource>
</testResources>
</build>
Karate Configuration File
In startup karate expects a java-script file called karate-config.js. In this file we can define configuration as a JSON object. All the keys and values in the JSON object will be made available as test scrip variables. For example we can save the baseUrl of the REST API. Create a file karete-config.js and place it src/test/java directory
function fn() { let config = { baseUrl : 'http://localhost:8080/v1/api' }; return config; }
First Test
We have the following mock API that create a employee include in our project.
The feature file is in plain English which is easy to understand what is happening, even non programmers can read and understand to some extend.Here the baseUrl is picked from karate-config.js file and json response is matched with the status code.
With IDE support in the development phase you can run these individual test by right click and run the feature file. We'll see later how to run all tests at once from the command line with junit.
Now we have few tests, we can run these tests at once by creating a java class and defining the tests in the class. Create EmployeeTest class in the same directory.
Now run mvn test in your terminal to run all the tests at once.
Url: http://localhost:8080/api/v1/employee Method: POST Request Body: { "id": [string], "name": [string] }Let's create a feature file create-employee.feature in /src/test/java/your-package/ directory.
The feature file is in plain English which is easy to understand what is happening, even non programmers can read and understand to some extend.Here the baseUrl is picked from karate-config.js file and json response is matched with the status code.
With IDE support in the development phase you can run these individual test by right click and run the feature file. We'll see later how to run all tests at once from the command line with junit.
With JUNIT 5
Above example has only one scenario, let's test another feature in this API. Create file get-employee.feature in the same directory with below content.Now we have few tests, we can run these tests at once by creating a java class and defining the tests in the class. Create EmployeeTest class in the same directory.
Now run mvn test in your terminal to run all the tests at once.
Match multiple responses data tables.
You send and match multiple requests response by simply using a data table. In the same create-employee feature file add the below scenario to use data table, here name from the request is mapped with name in the response.
Match response from json file
If you are getting a large payload as response, easiest way is to match response from a file. karate have a read function for this. You can read many files types in karate including json. Below example shows how to match a list of response returning back from your response. You can add the json file in the same directory alone side with your feature files.
Karate also support dynamic data testing, I will post about how to test with dynamic data in a future blog post.
Reporting
Since we added the maven-surfire-plugin, after the execution of each feature with JUnit a individual HTML report is generated in the /target/surfire-reports directory.
Cucumber Reporting
The default reports contains individual feature reporst, to get an overview report you can use cucumber reporting . Karate suggesting us to use cucumber-reporting library programmatically in combination with the Karate parallel runner. Use the below maven dependency.
<dependency> <groupId>net.masterthought</groupId> <artifactId>cucumber-reporting</artifactId> <version>3.8.0</version> <scope>test</scope> </dependency>We need to use karate parallel runner to run all the tests in parallel. We need to create CucumberReportParallelRunner class in the classpath. (/src/test/java)
To run and generate the report you can use the below maven command.
mvn -Dtest=CucumberReportParallelRunner testYou can find the generated report in target/cucumber-html-reports directory. Below is a cucumber report generate from this project.
![]() |
cucumber-report |
Conclusion
I hope you got basic the understanding karate and how to use it for your API testing. I will post more on karate features. Feel free to share your thoughts on this testing framework.
Happy Coding.
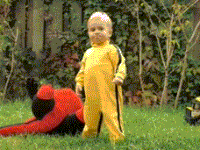
References & Useful Readings.
Good start with this framework. 👍
ReplyDeleteThank you very much for the feedback .
DeleteGreat article :)
ReplyDeleteThank you very much for reading my post !!!
DeleteGood content. Keep it up !
ReplyDeleteThank you very much wasantha
DeleteGood start , can you also for soap webservice automation using karate
ReplyDeleteHi Samfeep, we can use karate to test soap webservices, see the example from karate,
Deletehttps://github.com/intuit/karate/blob/master/karate-demo/src/test/java/demo/soap/soap.feature
Hi,
ReplyDeleteHow we add the mock server ???
Hi Punit, sorry for the late reply, The mock server is already their in the project, you just need to run the spring boot application
Deletehttps://github.com/Rajithkonara/API-test-karate-example
This comment has been removed by a blog administrator.
ReplyDeleteThe article you have shared here about this topic is really significant for us. I'm happy that you have shared this great info with us. Keep posting, Thank you. Fencing For Children in London
ReplyDeleteIt is truly a well-researched content and excellent wording. I got so engaged in this material that I couldn’t wait to read. I am impressed with your work and skill. Thanks. Read more info about Krav Maga Training Orlando
ReplyDelete