Photo credits : https://www.pexels.com/photo/close-up-photo-of-green-leafed-plants-1213859/
In this blog post let's see how we integrate and use Redis cache with spring boot application. I wanted to demonstrate a practical example here. Recently I had to work with a service that used to sent otp (one time password) to users to verify their mobile number and email. So to store the otp value with a key we had to use a cache.
To install redis follow the instructions - https://redis.io/download
These are the exact instruction given in the above link too
- Edit redis.conf, uncomment requirepass
eg: requirepass yourpassword
Now go to src directory and start the redis-server by typing
1) Set key (save to cache)
set test@gmail.com 00001
2) Get values
get test@gmail.com
You should be getting a example output as below. As you can see the values are saved as key value pairs, in the below example the key is the email address which is test@gmail.com and its value is 123456
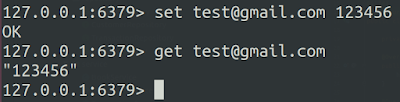
Now we are all set with the redis server, let's check out the code, I will be posting only the important classes here, you can find the Github link for the full code in the end of this blog post. To integrate redis with spring-boot, out of the box spring supports the redis integration with a redis client known as lettuce (https://lettuce.io/) . So to getting start you need to add below dependency to your pom.
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-redis</artifactId>
</dependency>
We are almost done with the redis configuration with spring-boot, now we can use the redis cache in out application. To demonstrate that we can use the redis cache, I have implemented simple controller which generate a OTP (one time password) to a given email address (our key). The generated OTP will be saved in the cache with the key as email address and value as the otp.
This key value pair will be saved in the redis cache.
Once we send a OTP verify request to verify the value, if the key and value we send is available in the cache, the key will be removed from the cache.
References & Useful Readings.
https://lettuce.io/
https://redis.io/
https://www.bytepitch.com/blog/redis-integration-spring-boot/
In this blog post let's see how we integrate and use Redis cache with spring boot application. I wanted to demonstrate a practical example here. Recently I had to work with a service that used to sent otp (one time password) to users to verify their mobile number and email. So to store the otp value with a key we had to use a cache.
To install redis follow the instructions - https://redis.io/download
These are the exact instruction given in the above link too
$ wget http://download.redis.io/releases/redis-5.0.7.tar.gz
$ tar xzf redis-5.0.7.tar.gz
$ cd redis-5.0.7
$ make
Adding password to redis server
- Go to redis-installation-dir- Edit redis.conf, uncomment requirepass
eg: requirepass yourpassword
Now go to src directory and start the redis-server by typing
./redis-server ../redis.conf
Since we have enabled password protection, to use cli you need to authenticate yourself. Start the redis cli by typing./redis-cli
Then authenticate by typingAUTH yourPassword
Sample redis commands1) Set key (save to cache)
set test@gmail.com 00001
2) Get values
get test@gmail.com
You should be getting a example output as below. As you can see the values are saved as key value pairs, in the below example the key is the email address which is test@gmail.com and its value is 123456
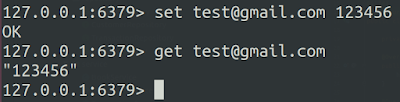
Now we are all set with the redis server, let's check out the code, I will be posting only the important classes here, you can find the Github link for the full code in the end of this blog post. To integrate redis with spring-boot, out of the box spring supports the redis integration with a redis client known as lettuce (https://lettuce.io/) . So to getting start you need to add below dependency to your pom.
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-redis</artifactId>
</dependency>
Configuring Redis
Let's create a configuration class to hold the redis configuration.
Now let's implement a CacheRepository to make operation on redis using the RedisTemplate. Here I have used the ValueOperation which can we simply worked with String values, for more different operations their are many Interfaces you can use like HashOperations for redis hash operations.ttl for cache
To set a ttl, (time to live) the time period that value is exists in the cache. We can set a ttl value for every key that stored in the cache. So after the ttl value has expired on a key, that key will be automatically removed from the cache. (See the line 41 in the OTPCacheRepository) In this example ttl value is defined as a config value in the application.yml file.We are almost done with the redis configuration with spring-boot, now we can use the redis cache in out application. To demonstrate that we can use the redis cache, I have implemented simple controller which generate a OTP (one time password) to a given email address (our key). The generated OTP will be saved in the cache with the key as email address and value as the otp.
This key value pair will be saved in the redis cache.
Once we send a OTP verify request to verify the value, if the key and value we send is available in the cache, the key will be removed from the cache.
Now check the redis-cli for the keys and you will see that the key is removed from the cache. Sample Postman collection is available in the repository. Source code can be found in below link.
https://lettuce.io/
https://redis.io/
https://www.bytepitch.com/blog/redis-integration-spring-boot/
Comments
Post a Comment